1st - this is NOT a full blown CNC lathe control!
This project has two modes of operation, drive the carriage using a stepper motor and display RPM/SFM. It is very helpful to me for reducing stock diameter, making sets of spacers all the same length and other operations.

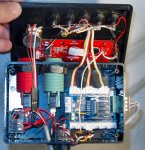
The first photo is of the control head. The button functions from left are:
Stop - in stepper mode stop moving the carriage, in RPM/SFM reset the workpiece diameter.
<< - in stepper mode move the carriage left or decrement carriage movement distance, in RPM/SFM decrement the workpiece diameter.
>> - in stepper mode move the carriage right or increment carriage movement distance, in RPM/SFM increment the workpiece diameter.
Prog Mode - (stepper mode only) step through the possible program modes: Free Run, Prog Left, Prog Right, Prog Left with Tool
Prog Run - (stepper mode only) start the selected program mode running.
The second photo shows the inside of the control box. This project uses a AXE401 board and a Seed Studio Motor Shield to minimize development time. All connections are out of the interfacing manual except for the large red & green buttons, the pot and relay on the left side. These control the lathe motor via a Variable Frequency Drive. Currently they have no connection to the Picaxe. In the future I will replace the micro switch on the big red stop button to include contacts to stop the stepper motor via the Picaxe.
The code is in multiple sections due to forum limitations.
This project has two modes of operation, drive the carriage using a stepper motor and display RPM/SFM. It is very helpful to me for reducing stock diameter, making sets of spacers all the same length and other operations.

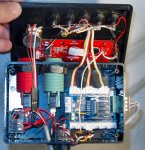
The first photo is of the control head. The button functions from left are:
Stop - in stepper mode stop moving the carriage, in RPM/SFM reset the workpiece diameter.
<< - in stepper mode move the carriage left or decrement carriage movement distance, in RPM/SFM decrement the workpiece diameter.
>> - in stepper mode move the carriage right or increment carriage movement distance, in RPM/SFM increment the workpiece diameter.
Prog Mode - (stepper mode only) step through the possible program modes: Free Run, Prog Left, Prog Right, Prog Left with Tool
Prog Run - (stepper mode only) start the selected program mode running.
The second photo shows the inside of the control box. This project uses a AXE401 board and a Seed Studio Motor Shield to minimize development time. All connections are out of the interfacing manual except for the large red & green buttons, the pot and relay on the left side. These control the lathe motor via a Variable Frequency Drive. Currently they have no connection to the Picaxe. In the future I will replace the micro switch on the big red stop button to include contacts to stop the stepper motor via the Picaxe.
The code is in multiple sections due to forum limitations.
Code:
# picaxe 28x2
' Metal lathe control and display program
' 2 modes of operation, drive the carriage using a stepper motor and display RPM/SFM
'
' Bipolar stepper motor lathe carriage feed
' Seeedstudio Arduino Motor Shield v1 (C.1 & C.2 held high to enable)
' Left and Right are as viewed from front of motor 1=Red(A),2=Blue(-A),3=Green(B),4=Black(-B)
' Left is toward the headstock
' 1000 steps = .076" (+-.0015") so 1 step = .000076275"
' Sparkfun ADM1602U 2x16 serial LCD
'
' Revolutions Per Minute / Surface Feet per Minute use a hall effect (US5881LUA) sensor
' SFM calculation uses a user entered work piece diameter
symbol LCDpin = A.1
symbol StopPin = pinA.0
symbol LeftPin = pinA.2
symbol RightPin = pinA.3
symbol ProgPin = pinB.3 'marked a4 on board
symbol RunPin = pinB.4 'marked a5 on board
symbol HallPin = B.0 'marked d3 on board - hall efect sensor
symbol ModePin = pinB.1 'marked d4 on board - RPM&SFM or stepper mode
symbol StepVar1 = b0 'counter for stepper motor subroutine
symbol StepVar2 = b1 'output var to set pins for stepper motor subroutine
symbol LoopVar1 = w1 'starting value for programmed run loops starts at 0
symbol LoopVar2 = w2 'ending value for programmed run loops in steps
symbol ProgVar1 = w3 'how far to run in program mode, (currently in .01" resolution?)
symbol SlopStep = w4 'number of steps to run when reversing direction
symbol ToolWidth= w5 'width of tool in steps
symbol StepCount= w6 'count of steps run in free run mode
symbol RPM = w7 'Revolutions Per Minute
symbol SFM = w8 'Surface Feet per Minute
symbol StepConst= w9 'thousandths per 1000 steps
symbol ProgMode = b20 '0=free run,1=prog left,2=prog right,3=prog left with tool,4=RPM/SFM
symbol RunDir = b21 '1=left 2=right
symbol Dis10000 = b22 'BinToASCII display variable
symbol Dis1000 = b23 'BinToASCII display variable
symbol Dis100 = b24 'BinToASCII display variable
symbol Dis10 = b25 'BinToASCII display variable
symbol Dis1 = b26 'BinToASCII display variable
symbol FirstRun = b27 'skip ProgMode 4 and above after first loop
symbol ModeChng = b28 'detect change of mode from RPM/SFM vs stepper mode
symbol Dia = w15 'diameter for SFM calc, 0.1 resolution
symbol Mark = w16 'mark time of hall sensor for RPM calc
symbol Space = w17 'space time of hall sensor for RPM calc
'used for double word division
symbol topbit = $8000 ; Value of "carry" bit (when shifting left)
symbol pass = b49 ; Loop counter, on return = 0 if data error
symbol numhi = w25 ; Numerator Hi, Returns Remainder
symbol numlo = w26 ; Numerator Lo, Retuns Result
symbol divis = w27 ; Divisor (max 15 bits), Unchanged on Return
ProgVar1 = 30 'starting value for programed runs (0.030)
ProgMode = 0 '0=free run,1=prog left,2=prog right,3=prog left with tool
SlopStep = 734 'number of steps to run when reversing direction
ToolWidth= 725 'steps for tool width (725 for .0625 cutoff)
StepConst= 76 'steps per 0.001
FirstRun = 0 'allow changes to SlopStep, ToolWidth & StepConst on first run through ProgMode
ModeChng = 0 'refresh LCD when stepper vs RPM/SFM mode changes
Dia = 500 'starting value for diameter for SFM calc (0.200)
dirsC = %11111111 'setup output pins for stepper motor
pause 1000
serout LCDpin, t9600_8,(254,124,"<control>r")
serout LCDpin, t9600_8,(254,128,"Stepper14.bas ")
serout LCDpin, t9600_8,(254,192,"LG 07/02/2013 ")
pause 5000
serout LCDpin, t9600_8,(254,128,"Free Run Mode ")
serout LCDpin, t9600_8,(254,192,"Ready ")
main:
if ModePin = 0 then 'stepper control
if ModeChng = 1 then 'refresh LCD when changing from RPM/SFM mode
serout LCDpin, t9600_8,(254,124,"<control>r")
gosub DisplayProgMode
ModeChng = 0
end if
if LeftPin = 1 then 'pin starts free run left AND increments ProgVar1 depending on ProgMode
pause 200
if ProgMode = 0 then
StepCount = 0
serout LCDpin, t9600_8,(254,192,"Running Left ")
do
gosub lstep
StepCount = StepCount + 1
if StopPin = 1 then
BinToASCII StepCount,Dis10000,Dis1000,Dis100,Dis10,Dis1
serout LCDpin, t9600_8,(254,192,"Stop ",Dis10000,Dis1000,Dis100,Dis10,Dis1," steps")
exit
end if
loop
RunDir = 1
else if ProgMode > 0 then
if ProgVar1 > 1 then
ProgVar1 = ProgVar1 - 1
end if
BinToASCII ProgVar1,Dis10000,Dis1000,Dis100,Dis10,Dis1
if ProgMode = 1 then
serout LCDpin, t9600_8,(254,128,"Prog Left: ",Dis1000,".",Dis100,Dis10,Dis1)
else if ProgMode = 2 then
serout LCDpin, t9600_8,(254,128,"Prog Right:",Dis1000,".",Dis100,Dis10,Dis1)
else if ProgMode = 3 then
serout LCDpin, t9600_8,(254,128,"P L w/tool:",Dis1000,".",Dis100,Dis10,Dis1)
end if
end if
end if
if RightPin = 1 then 'pin starts free run right AND decrements ProgVar1 depending on ProgMode
pause 200
if ProgMode = 0 then
StepCount = 0
serout LCDpin, t9600_8,(254,192,"Running Right ")
do
gosub rstep
StepCount = StepCount + 1
if StopPin = 1 then
BinToASCII StepCount,Dis10000,Dis1000,Dis100,Dis10,Dis1
serout LCDpin, t9600_8,(254,192,"Stop ",Dis10000,Dis1000,Dis100,Dis10,Dis1," steps")
exit
end if
loop
RunDir = 2
else if ProgMode > 0 then
ProgVar1 = ProgVar1 + 20
BinToASCII ProgVar1,Dis10000,Dis1000,Dis100,Dis10,Dis1
if ProgMode = 1 then
serout LCDpin, t9600_8,(254,128,"Prog Left: ",Dis1000,".",Dis100,Dis10,Dis1)
else if ProgMode = 2 then
serout LCDpin, t9600_8,(254,128,"Prog Right:",Dis1000,".",Dis100,Dis10,Dis1)
else if ProgMode = 3 then
serout LCDpin, t9600_8,(254,128,"P L w/tool:",Dis1000,".",Dis100,Dis10,Dis1)
end if
end if
end if
if ProgPin = 1 then 'pin increments ProgMode variable
pause 500
if FirstRun = 0 then
if ProgMode < 6 then
ProgMode = ProgMode + 1
else
ProgMode = 0
FirstRun = 1
end if
else if FirstRun = 1 then
if ProgMode < 3 then
ProgMode = ProgMode + 1
else
ProgMode = 0
end if
end if
gosub DisplayProgMode 'refresh LCD
end if
'*** CONTINUED NEXT POST ***
Last edited: