Jeremy Harris
Senior Member
I've knocked up a simple circuit to control a BLDC motor controller. It does two things, it converts a throttle pot to the right voltage range for the controller throttle input and it has an auto-park capability to detect the position of the motor shaft (using a Hall sensor switch) and turn the power off when the shaft has motored (slowly) to the right position (it's for a boat, where I want the two blade prop to be vertical when the power is turned off).
The first version used c.3 as the power switch input (S1 in the drawing) and c.5 as the shaft position switch input (S2 in the drawing, both are active low). This didn't work, with the code only working after the shaft had been rotated by hand until c.5 went low. For some reason the state of c.3 was ignored until c.5 had been taken low, then it worked normally.
The final version (code attached) has the functions of c.3 and c.5 reversed and works as designed.
It's not a particular problem (although it has had me scratching my head for an hour or two) but can anyone see why reading the state of c.3 was ignored when I had that as the "power on" (active low) switch input?
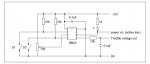
The first version used c.3 as the power switch input (S1 in the drawing) and c.5 as the shaft position switch input (S2 in the drawing, both are active low). This didn't work, with the code only working after the shaft had been rotated by hand until c.5 went low. For some reason the state of c.3 was ignored until c.5 had been taken low, then it worked normally.
The final version (code attached) has the functions of c.3 and c.5 reversed and works as designed.
It's not a particular problem (although it has had me scratching my head for an hour or two) but can anyone see why reading the state of c.3 was ignored when I had that as the "power on" (active low) switch input?
Code:
;This code works with c.3 and c.5 swapped over but doesn't work with c.3 as the power switch input and c.5 as the shaft position switch input - don't know why
SYMBOL Throttle = w0
#picaxe 08m2
init:
DISCONNECT ;disable serial input scanning on pin c.5
main:
IF pinc.5 = 0 THEN GOTO running ;power switch state check and run normally
IF pinc.5 = 1 AND b2 = 0 THEN GOTO park ;power switch state check and park flag check - park prop if not already parked
IF pinc.5 = 1 AND b2 = 1 THEN GOTO stopped ;power switch state check and park flag check - turn off controller if already parked
HIGH c.1 ;error trap - turn off controller and return back to this loop
GOTO main
running:
LOW c.1 ;turn on controller power
READADC10 c.4,Throttle ;read throttle setting from throttle input and scale for controller output range (input range is 0 to 1023)
Throttle = Throttle * 10 ;multiply to allow math for scaling
Throttle = Throttle / 53 ;scale to give max throttle voltage of 3.4 V
Throttle = Throttle + 82 ;add 1 V offset to remove dead period from throttle at start
PWMOUT c.2, 99, Throttle ;99 sets 10kHz on timer, max duty cycle range on PWM is 0 to 400
b2 = 0 ;reset parked flag to un-parked
GOTO main
park:
Throttle = 120
PWMOUT c.2, 99, Throttle ;set slow speed to rotate prop to correct position
park1:
IF pinc.3 = 1 THEN GOTO park1 ;check for Hall active, loop quickly to detect parked position
HIGH c.1 ;turn off controller and set throttle to zero
Throttle = 0
PWMOUT c.2, 99, Throttle
b2 = 1 ;set parked flag
GOTO main
stopped:
HIGH c.1 ;turn off controller and set throttle to zero
Throttle = 0
PWMOUT c.2, 99, Throttle
GOTO main
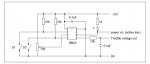