My stepper kits arrived from DX: http://www.dealextreme.com/p/28ybt-48-stepper-motor-with-uln2003-driver-dc-5v-126409?item=2
This is how the board is wired:
Seem to work fine. I'm driving it at 6v and it's drawing <300mA with no load.
This is the test code I'm using:
It rotates 360 degrees CW then 360 degrees CCW and repeats. The 5 bits instead of 4 in the lookup is because pinC.3 cannot be an output on the 08m2, so I have to use pinC.4 for the fourth bit.
Question. How can you tell when a step has been missed/slipped due to excessive drag on the spindle?
Reason I ask is coz I might use this kit to make an avionics-type heading indicator for my little car using a magnetometer and a stepper to rotate the disk. The stepper can sync/calibrate for absolute position on startup using maybe an optical interrupter thingy. But from then on, I want it to remain in sync without further calibration for hours at a time, keeping true to the commanded compass direction.
This is how the board is wired:

Seem to work fine. I'm driving it at 6v and it's drawing <300mA with no load.
This is the test code I'm using:
Code:
#Picaxe 08m2
'Stepper motor driver test
'Boris Burke May 2012
setfreq m8
dirs=%00010111
do
b1=b1+2 max 3//3 'b1 is direction control, 2=CW 0=CCW
for w1=1 to 2060 'seems to be about the right number of steps for 360 degrees
b0=b0+b1-1//4 'b0 cycles from 0 to 3 for CW and from 3 to 0 for CCW
lookup b0,(%00011,%00110,%10100,%10001),pinsC
pause 1
next w1
pause 4000
loop
Question. How can you tell when a step has been missed/slipped due to excessive drag on the spindle?
Reason I ask is coz I might use this kit to make an avionics-type heading indicator for my little car using a magnetometer and a stepper to rotate the disk. The stepper can sync/calibrate for absolute position on startup using maybe an optical interrupter thingy. But from then on, I want it to remain in sync without further calibration for hours at a time, keeping true to the commanded compass direction.
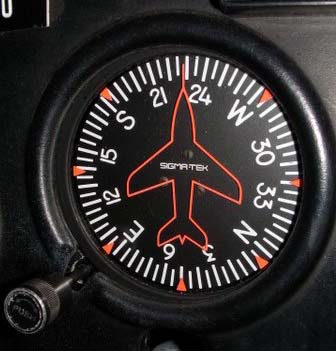
Last edited: