rq3
Senior Member
Merry Christmas, all. This is only my second Picaxe project. The first was very satisfying, and I'm trying to play Apollo era NASA engineer on this one to strip the code as severely as possible. I would appreciate tremendously any and all comments on this code. My intent is for it to run as fast as possible, and I have tried subroutines, select case, and multiple "Starts". This seems to be the best I can do. I know, I know, it's simple and primitive, but the fun
is in the effort (and the fabrication of the board, and the testing, and...).
I've attempted to incorporate previous suggestions as to editing, indenting, use of symbols, etc. Fire at will, or at me if Will is not available.
Schematic:
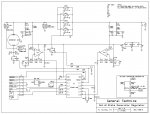
is in the effort (and the fabrication of the board, and the testing, and...).
I've attempted to incorporate previous suggestions as to editing, indenting, use of symbols, etc. Fire at will, or at me if Will is not available.
Schematic:
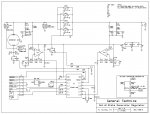
Code:
#rem
*******************************************************
* Code for a Delco-Remy Type A generator regulator. The PICAXE 14M2 *
* does a 10 bit A/D conversion of the generator voltage at Pin C.0, *
* and a 10 bit A/D conversion of the battery voltage at Pin B.5. *
* After voltage division by 5.72, op-amps are used to provide a non- *
* inverting gain of 9.53 and offset such that the A/D inputs are 0-5 *
* volts over a battery voltage of 12.8 VDC to 15.8 VDC. A nominal *
* 14.3 VDC battery charge (V_Set)results in an A/D conversion output *
* word value of 512, programmable at 3mV per bit. Battery voltage *
* under the setpoint causes the PICAXE to turn on the generator field. *
* Generator voltage higher than the battery voltage causes the PICAXE *
* to turn on paralleled P channel reverse biased MOSFETS, which act as *
* reverse current relays. Under-voltage and over-voltage conditions *
* detected by the PICAXE illuminate appropriate LEDs, and excessive *
* voltage drop across the P channel MOSFETs is used to flag a high *
* current condition from the generator and turn off its field. *
*******************************************************
#endrem
#PICAXE14M2 ;initialize for 14M2
setfreq m32 ;set clock to 32 MHz
symbol V_Set=512 ;charge voltage at +14.3VDC
symbol Bat=w0
symbol Gen=w1
symbol Cur=w2
let Cur=w1-w0
Main:
readadc10 B.5,Bat ;read the battery voltage
readadc10 C.0,Gen ;read the generator voltage
if Bat<V_Set then high B.1 ;if bat<setpoint, turn on field
else low B.1
endif
if Gen>Bat then high B.2 ;if gen>bat,turn on P-Ch MOSFETs
else low B.2
endif
if Bat<10 then high C.1 ;if bat<12.8 volt,
low C.2 ;turn on LED D5 (undervoltage)
low C.1
endif
if Bat>1000 then low C.1 ;if bat>15.8 volt
low B.1 ;turn off field
high C.2 ;turn on LED D4 (overvoltage)
high C.1
endif
if Cur>20 then low B.1 ;turn off field if current is excessive
endif
goto Main
Attachments
-
2.2 KB Views: 8